Standalone Spring applications
last modified July 13, 2020
In this tutorial, we are going to create two simple Java Spring standalone applications. We will use NetBeans to build the applications.
Spring is a popular Java application framework. It provides various libraries and tools for enterprise application programming. It is also a very good integration system that helps glue together various enterprise components.
Spring ApplicationContext
is a central interface to provide
configuration for an application. ClassPathXmlApplicationContext
is
an implementation of the ApplicationContext
that loads
configuration definition from an XML file, which is located on the classpath.
AnnotationConfigApplicationContext
creates a new application
context deriving bean definitions from the given annotated classes.
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.zetcode</groupId> <artifactId>SpringStandaloneEx2</artifactId> <version>1.0-SNAPSHOT</version> <packaging>jar</packaging> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> <spring-version>4.3.0.RELEASE</spring-version> </properties> <dependencies> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring-version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-beans</artifactId> <version>${spring-version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>${spring-version}</version> </dependency> </dependencies> </project>
We use this Maven build file for both applications. It contains the necessary Spring dependencies.
Spring application with ClassPathXmlApplicationContext
We create a new Maven Java SE application in NetBeans IDE.
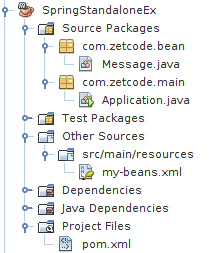
In the project, there are four files: Message.java
, Application.java
,
my-beans.xml
, and pom.xml
.
package com.zetcode.bean; public class Message { private String message; public void setMessage(String message){ this.message = message; } public String getMessage(){ return message; } }
Message
is a simple Java bean used in our application.
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <bean id="mymessage" class="com.zetcode.bean.Message"> <property name="message" value="Hello there!"/> </bean> </beans>
We make the Message
class into a Spring bean; it is
now managed by the Spring container. We also provide a value
for the message
property. The my-beans.xml
is
located in the src/main/resources
subdirectory.
package com.zetcode.main; import com.zetcode.bean.Message; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class Application { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("my-beans.xml"); Message obj = (Message) context.getBean("mymessage"); String msg = obj.getMessage(); System.out.println(msg); } }
The Application
sets up the Spring application.
ApplicationContext context = new ClassPathXmlApplicationContext("my-beans.xml");
From the my-beans.xml
file, we create the ApplicationContext
.
Message obj = (Message) context.getBean("mymessage");
From the application context, we retrieve the Message
bean.
String msg = obj.getMessage(); System.out.println(msg);
We call the bean's getMessage
method and print the message to
the console.
Hello there!
This is the output of the application.
Spring application with AnnotationConfigApplicationContext
In the second example, we are going to use a AnnotationConfigApplicationContext
to create the Spring ApplicationContext
.
package com.zetcode.bean; import org.springframework.stereotype.Component; @Component public class Message { private String message = "Hello there!"; public void setMessage(String message){ this.message = message; } public String getMessage(){ return message; } }
The Message
bean is decorated with the @Component
annotation.
Such classes are auto-detected by Spring.
package com.zetcode.main; import com.zetcode.bean.Message; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.context.ApplicationContext; import org.springframework.context.annotation.AnnotationConfigApplicationContext; import org.springframework.context.annotation.ComponentScan; @ComponentScan(basePackages = "com.zetcode") public class Application { public static void main(String[] args) { ApplicationContext context = new AnnotationConfigApplicationContext(Application.class); Application p = context.getBean(Application.class); p.start(); } @Autowired private Message message; private void start() { System.out.println("Message: " + message.getMessage()); } }
This is the main Application
class.
@ComponentScan(basePackages = "com.zetcode")
With the @ComponentScan
annotation we tell Spring where to look
for components.
ApplicationContext context = new AnnotationConfigApplicationContext(Application.class);
The ApplicationContext
is created from annotations.
@Autowired private Message message; private void start() { System.out.println("Message: " + message.getMessage()); }
With the @Autowired
annotation, the Message
bean is
injected into the message
variable.
In this tutorial, we have created two standalone Spring application. The first one was using an XML file, the second one relied on annotations.