Events in wxWidgets
last modified October 18, 2023
Events are integral part of every GUI application. All GUI applications are event-driven. An application reacts to different event types which are generated during its lifetime. Events are generated mainly by the user of an application. But they can be generated by other means as well, e.g. an Internet connection, a window manager, or a timer. When the application starts a main loop is created. The application sits in the main loop and waits for the events to be generated. The main loop quits, when we exit the application.
Definitions
Event is a piece of application-level information from the underlying framework, typically the GUI toolkit. Event loop is a programming construct that waits for and dispatches events or messages in a program. The event loop repeatedly looks for events to process them. A dispatcher is a process which maps events to event handlers. Event handlers are methods that react to events.
Event object is an object associated with the event. It is usually a window. Event type is a unique event that has been generated.
A simple event example
The traditional way to work with events in wxWidgets is to use
static event tables. This was influenced by the Microsoft
Foundation Classes (MFC). A more flexible and modern way is to use the
Connect
method. We use it throughout the wxWidgets tutorial.
Event table
In the next example, we show an example, where we use event tables.
#include <wx/wx.h> class MyButton : public wxFrame { public: MyButton(const wxString& title); void OnQuit(wxCommandEvent& event); private: DECLARE_EVENT_TABLE() };
#include "button.h" MyButton::MyButton(const wxString& title) : wxFrame(NULL, wxID_ANY, title, wxDefaultPosition, wxSize(270, 150)) { wxPanel *panel = new wxPanel(this, wxID_ANY); wxButton *button = new wxButton(panel, wxID_EXIT, wxT("Quit"), wxPoint(20, 20)); Centre(); } void MyButton::OnQuit(wxCommandEvent& WXUNUSED(event)) { Close(true); } BEGIN_EVENT_TABLE(MyButton, wxFrame) EVT_BUTTON(wxID_EXIT, MyButton::OnQuit) END_EVENT_TABLE()
#include <wx/wx.h> class MyApp : public wxApp { public: virtual bool OnInit(); };
#include "main.h" #include "button.h" IMPLEMENT_APP(MyApp) bool MyApp::OnInit() { MyButton *button = new MyButton(wxT("Button")); button->Show(true); return true; }
In our example we create a simple button. By clicking on the button, we close the application.
private: DECLARE_EVENT_TABLE()
In the header file, we declare an event table with the
DECLARE_EVENT_TABLE
macro.
BEGIN_EVENT_TABLE(MyButton, wxFrame) EVT_BUTTON(wxID_EXIT, MyButton::OnQuit) END_EVENT_TABLE()
We implement an event table by mapping each event to the appropriate member function.
Example using Connect()
We talk about a move event. A move event holds information about
move change events. A move event is generated, when we move a window to
a new position. The class that represents the move event is wxMoveEvent
.
The wxEVT_MOVE
is an event type.
#include <wx/wx.h> class Move : public wxFrame { public: Move(const wxString& title); void OnMove(wxMoveEvent & event); wxStaticText *st1; wxStaticText *st2; };
#include "move.h" Move::Move(const wxString& title) : wxFrame(NULL, wxID_ANY, title, wxDefaultPosition, wxSize(250, 130)) { wxPanel *panel = new wxPanel(this, -1); st1 = new wxStaticText(panel, -1, wxT(""), wxPoint(10, 10)); st2 = new wxStaticText(panel, -1, wxT(""), wxPoint(10, 30)); Connect(wxEVT_MOVE, wxMoveEventHandler(Move::OnMove)); Centre(); } void Move::OnMove(wxMoveEvent& event) { wxPoint size = event.GetPosition(); st1->SetLabel(wxString::Format(wxT("x: %d"), size.x )); st2->SetLabel(wxString::Format(wxT("y: %d"), size.y )); }
#include <wx/wx.h> class MyApp : public wxApp { public: virtual bool OnInit(); };
#include "main.h" #include "move.h" IMPLEMENT_APP(MyApp) bool MyApp::OnInit() { Move *move = new Move(wxT("Move event")); move->Show(true); return true; }
The example displays the current position of the window.
Connect(wxEVT_MOVE, wxMoveEventHandler(Move::OnMove));
Here we connect a wxEVT_MOVE
event type with the
OnMove
method.
wxPoint size = event.GetPosition();
The event parameter in the OnMove
method is an object specific
to a particular event. In our case it is the instance of a wxMoveEvent
class.
This object holds information about the event. We can find out the current position
by calling the GetPosition
method of the event.
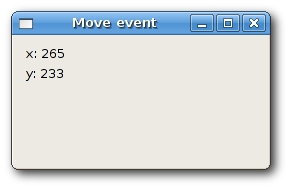
Event propagation
There are two types of events: basic events and command events. They differ
in propagation. Event propagation is traveling of events from child widgets
to parent widgets and grand parent widgets etc. Basic events do not propagate.
Command events do propagate. For example wxCloseEvent
is a basic event.
It does not make sense for this event to propagate to parent widgets.
By default, the event that is caught in a event handler stops propagating.
To continue propagation, we must call the Skip
method.
#include <wx/wx.h> class Propagate : public wxFrame { public: Propagate(const wxString& title); void OnClick(wxCommandEvent& event); }; class MyPanel : public wxPanel { public: MyPanel(wxFrame *frame, int id); void OnClick(wxCommandEvent& event); }; class MyButton : wxButton { public: MyButton(MyPanel *panel, int id, const wxString &label); void OnClick(wxCommandEvent& event); };
#include <iostream> #include "propagate.h" const int ID_BUTTON = 1; Propagate::Propagate(const wxString& title) : wxFrame(NULL, wxID_ANY, title, wxDefaultPosition, wxSize(250, 130)) { MyPanel *panel = new MyPanel(this, -1); new MyButton(panel, ID_BUTTON, wxT("Ok")); Connect(ID_BUTTON, wxEVT_COMMAND_BUTTON_CLICKED, wxCommandEventHandler(Propagate::OnClick)); Centre(); } void Propagate::OnClick(wxCommandEvent& event) { std::cout << "event reached frame class" << std::endl; event.Skip(); } MyPanel::MyPanel(wxFrame *frame, int id) : wxPanel(frame, id) { Connect(ID_BUTTON, wxEVT_COMMAND_BUTTON_CLICKED, wxCommandEventHandler(MyPanel::OnClick)); } void MyPanel::OnClick(wxCommandEvent& event) { std::cout << "event reached panel class" << std::endl; event.Skip(); } MyButton::MyButton(MyPanel *mypanel, int id, const wxString& label) : wxButton(mypanel, id, label, wxPoint(15, 15)) { Connect(ID_BUTTON, wxEVT_COMMAND_BUTTON_CLICKED, wxCommandEventHandler(MyButton::OnClick)); } void MyButton::OnClick(wxCommandEvent& event) { std::cout << "event reached button class" << std::endl; event.Skip(); }
#include <wx/wx.h> class MyApp : public wxApp { public: virtual bool OnInit(); };
#include "main.h" #include "propagate.h" IMPLEMENT_APP(MyApp) bool MyApp::OnInit() { Propagate *prop = new Propagate(wxT("Propagate")); prop->Show(true); return true; }
In our example, we have a button on a panel. The panel is placed in a frame widget. We define a handler for all widgets.
event reached button class event reached panel class event reached frame class
We get this, when we click on the button. The event travels from the button to panel and to frame.
Try to omit some Skip
methods and see what happens.
Vetoing events
Sometimes we need to stop processing an event. To do this,
we call the method Veto
.
#include <wx/wx.h> class Veto : public wxFrame { public: Veto(const wxString& title); void OnClose(wxCloseEvent& event); };
#include "veto.h" Veto::Veto(const wxString& title) : wxFrame(NULL, wxID_ANY, title, wxDefaultPosition, wxSize(250, 130)) { Connect(wxEVT_CLOSE_WINDOW, wxCloseEventHandler(Veto::OnClose)); Centre(); } void Veto::OnClose(wxCloseEvent& event) { wxMessageDialog *dial = new wxMessageDialog(NULL, wxT("Are you sure to quit?"), wxT("Question"), wxYES_NO | wxNO_DEFAULT | wxICON_QUESTION); int ret = dial->ShowModal(); dial->Destroy(); if (ret == wxID_YES) { Destroy(); } else { event.Veto(); } }
#include <wx/wx.h> class MyApp : public wxApp { public: virtual bool OnInit(); };
#include "main.h" #include "veto.h" IMPLEMENT_APP(MyApp) bool MyApp::OnInit() { Veto *veto = new Veto(wxT("Veto")); veto->Show(true); return true; }
In our example, we process a wxCloseEvent
. This event is called,
when we click the X button on the titlebar, press Alt+F4 or select
close from the system menu. In many applications, we want to prevent
from accidentally closing the window if we made some changes. To do
this, we must connect the wxEVT_CLOSE_WINDOW
event type.
wxMessageDialog *dial = new wxMessageDialog(NULL, wxT("Are you sure to quit?"), wxT("Question"), wxYES_NO | wxNO_DEFAULT | wxICON_QUESTION);
During the close event, we show a message dialog.
if (ret == wxID_YES) { Destroy(); } else { event.Veto(); }
Depending on the return value, we destroy the window, or veto the
event. Notice that to close the window, we must call the Destroy
method. By calling the Close
method, we would end up in an
endless cycle.
Window identifiers
Window identifiers are integers that uniquely determine the window identity in the event system. There are three ways to create a window id:
- Let the system automatically create an id.
- Use standard identifiers.
- Create our own id.
Each widget has an id parameter. This is a unique number in the event system. If we work with multiple widgets, we must differantiate among them.
wxButton(parent, -1) wxButton(parent, wxID_ANY)
If we provide -1 or wxID_ANY
for the ID parameter, we let wxWidgets
automatically create an id for us. The automatically created id's are
always negative, whereas user specified IDs must always be positive.
We usually use this option when we do not need to change the widget state.
For example a static text that will never be changed during the life of
the application. We can still get the ID if we want. There is a method
GetId
, which will determine the id for us.
Standard identifiers should be used whenever possible. The identifiers can provide some standard graphics or behaviour on some platforms.
#include <wx/wx.h> class Ident : public wxFrame { public: Ident(const wxString& title); };
#include "ident.h" Ident::Ident(const wxString& title) : wxFrame(NULL, wxID_ANY, title, wxDefaultPosition, wxSize(200, 150)) { wxPanel *panel = new wxPanel(this, -1); wxGridSizer *grid = new wxGridSizer(2, 3); grid->Add(new wxButton(panel, wxID_CANCEL), 0, wxTOP | wxLEFT, 9); grid->Add(new wxButton(panel, wxID_DELETE), 0, wxTOP, 9); grid->Add(new wxButton(panel, wxID_SAVE), 0, wxLEFT, 9); grid->Add(new wxButton(panel, wxID_EXIT)); grid->Add(new wxButton(panel, wxID_STOP), 0, wxLEFT, 9); grid->Add(new wxButton(panel, wxID_NEW)); panel->SetSizer(grid); Centre(); }
#include <wx/wx.h> class MyApp : public wxApp { public: virtual bool OnInit(); };
#include "main.h" #include "ident.h" IMPLEMENT_APP(MyApp) bool MyApp::OnInit() { Ident *ident = new Ident(wxT("Identifiers")); ident->Show(true); return true; }
In our example we use standard identifiers on buttons. On Linux, the buttons have small icons.
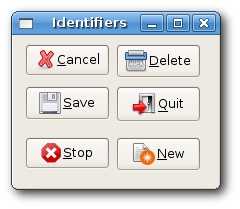
In this chapter, we talked about events in wxWidgets.