Document.querySelector tutorial
last modified October 18, 2023
In this article we show how to use querySelector
to
select HTML elements in JavaScript.
Document.querySelector
The Document's querySelector
method returns the first Element
within the document that matches the specified selector or a group of selectors.
If no matches are found, null is returned.
The querySelectorAll
returns a static NodeList representing a
list of the document's elements that match the specified group of selectors.
Document.querySelector example
The following example demonstrates the usage of querySelector
and querySelectorAll
methods.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document.querySelector</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/semantic-ui/2.3.1/components/button.min.css"> <style> body { margin: 3em } .selected { background-color: #eee } .container { display: grid; grid-template-columns: 100px 100px 100px 100px 100px; grid-template-rows: 50px; grid-column-gap:5px; margin-bottom: 1em; } div>div { border: 1px solid #ccc; } </style> </head> <body> <div class="container"> <div></div> <div></div> <div></div> <div></div> <div></div> </div> <button id="first" type="submit" class="ui grey button">First</button> <button id="all" type="submit" class="ui brown button">All</button> <button id="clear" type="submit" class="ui brown button">Clear</button> <script src="main.js"></script> </body> </html>
In the document, we display five bordered div elements. We have three buttons. Each button changes the look of the divs.
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/semantic-ui/2.3.1/components/button.min.css">
We style the document with Semantic UI.
.selected { background-color: #eee }
The selected divs have gray background colour.
.container { display: grid; grid-template-columns: 100px 100px 100px 100px 100px; grid-template-rows: 50px; grid-column-gap:5px; margin-bottom: 1em; }
The divs are displayed in one line using CSS grid layout system.
div>div { border: 1px solid #ccc; }
The divs within a container div have gray borders.
<div class="container"> <div></div> <div></div> <div></div> <div></div> <div></div> </div>
There are five divs placed in a parent container div.
<button id="first" type="submit" class="ui grey button">First</button> <button id="all" type="submit" class="ui brown button">All</button> <button id="clear" type="submit" class="ui brown button">Clear</button>
We have three buttons in the document. The First button changes the background colour of the first inner div. The All button changes all the inner divs. And the Clear button clears the backround of the divs. The buttons have Semantic UI styles.
<script src="main.js"></script>
The JavaScript code is placed in the main.js
file.
document.getElementById("first").onclick = (e) => { let tag = document.querySelector(".container div:first-child"); tag.className = "selected"; }; document.getElementById("all").onclick = (e) => { let tags = document.querySelectorAll(".container div"); tags.forEach( tag => { tag.className = "selected"; }); }; document.getElementById("clear").onclick = (e) => { let tags = document.querySelectorAll(".container div"); tags.forEach( tag => { tag.classList.remove("selected"); }); };
In the main.js
file, we implement the functionality of the
buttons.
document.getElementById("first").onclick = (e) => {
A click listener is added to the button with onclick
property.
The button is selected with getElementById
.
let tag = document.querySelector(".container div:first-child");
With the querySelector
method, we choose the first inner div within
the container div.
tag.className = "selected";
We add the selected
class to the chosen tag.
let tags = document.querySelectorAll(".container div");
With querySelectorAll
we choose all the inner divs.
tags.forEach( tag => { tag.className = "selected"; });
In a forEach
loop, we go through the list and append the class to
each of the elements.
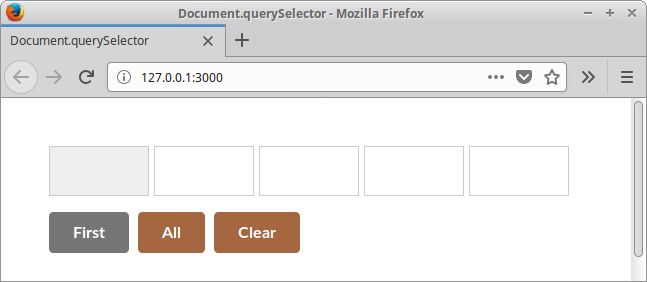
In the screenshot, we can see that the first div has its background colour changed.
Source
Document: querySelector method
In this article we have worked with querySelector
and
querySelectorAll
methods.